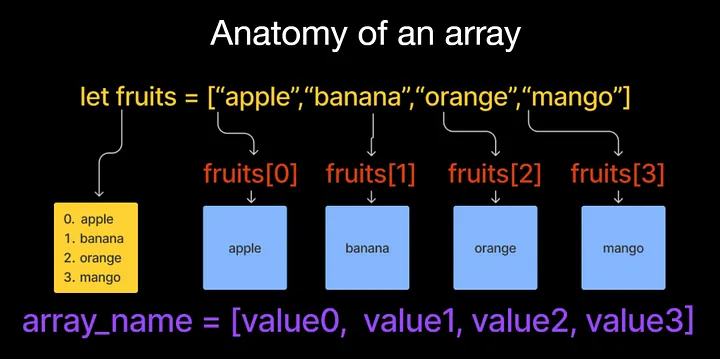
An array is a collection of items that are stored in a specific order. Each item in an array is called an element, and each element has a unique index that can be used to access it. Here is an example of an array in JavaScript:
var fruits = ["apple", "banana", "orange", "mango"];
In this example, we have created an array called fruits
that contains 4 elements: “apple”, “banana”, “orange”, and “mango”. The elements are stored in the order they are listed, and they are separated by commas.
You can access any element of the array by its index, which is a number that starts at 0. For example, to access the first element of the fruits array, you would use the index 0 like this:
console.log(fruits[0]); // Output: "apple"
You can also change the value of an element by using its index:
fruits[1] = "pineapple";
console.log(fruits); // Output: ["apple", "pineapple", "orange", "mango"]
You can also add new elements to the array by using the push() method:
fruits.push("kiwi");
console.log(fruits); // Output: ["apple", "pineapple", "orange", "mango", "kiwi"]
You can also remove elements from the array by using the pop() or splice() method:
fruits.pop(); // removes the last element of the array
console.log(fruits); // Output: ["apple", "pineapple", "orange", "mango"]
fruits.splice(1, 1); // removes the element at index 1
console.log(fruits); // Output: ["apple", "orange", "mango"]
These are just a few examples of how you can work with arrays in JavaScript. There are many other built-in methods and properties available for manipulating and working with arrays, such as sorting, filtering, and looping through the elements.
Arrays are a fundamental data structure in programming languages, and they are incredibly useful for storing and manipulating large sets of data.
One of the main reasons why arrays are so important in programming is that they allow you to store a large amount of data in a single variable. Instead of having to create a separate variable for each piece of data, you can store all of the data in an array and access it using the element’s index. This can make your code more organized and easier to read.
Another important feature of arrays is that they provide a way to iterate through the elements of the array. This is done by using a loop, such as a for loop, to access each element in the array and perform an action on it. This allows you to perform the same action on multiple elements at once, which can be more efficient and save you a lot of time.
Iterating through an array in JavaScript is a very common task, and it can be done using different types of loops such as for, forEach, map, and filter. Here we will see a simple example of how to use the for loop to iterate through an array and perform an action on each element.
// Declare an array
var fruits = ["apple", "banana", "orange", "mango"];
// Use a for loop to iterate through the array
for (var i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
In this example, we have an array called fruits
that contains 4 elements: “apple”, “banana”, “orange”, and “mango”. The for loop starts with the variable i
being set to 0. It will then run the loop until i
is no longer less than the length of the fruits
array. The i
variable is incremented by 1 on each iteration of the loop, so it will eventually reach the end of the array. Inside the loop, we’re using console.log
to print each element of the array.
The output will be:
apple
banana
orange
mango
The output will be the same as previous example.
It’s important to note that in these examples, the variable i
is used as the index to access the current element of the array. It’s also possible to use a for of loop in javascript to iterate through arrays.
for (const fruit of fruits) {
console.log(fruit);
}
It’s a simple and easy way to access the elements of an array, but it only works with arrays, it does not work with objects.
By understanding these simple examples you can start to see the power of arrays and loops in JavaScript, and how they can be used to make your code more efficient and organized. It’s also worth noting that these examples are just the tip of the iceberg when it comes to working with arrays in JavaScript, and there are many more advanced features and methods that can be used to manipulate and work with arrays.
In conclusion, arrays are an essential data structure in programming languages because they allow you to store and manipulate large sets of data in an organized and efficient manner. By using arrays, you can write more efficient and organized code, and solve problems more effectively.
Hello, I’m Anuj. I make and teach software.
My website is free of advertisements, affiliate links, tracking or analytics, sponsored posts, and paywalls.
Follow me on LinkedIn, X (twitter) to get timely updates when I post new articles.
My students are the reason this website exists. ❤️
Clear lessons, easy to follow even for a beginner. Very knowledgeable and gives great encouragement to beginner coders. Really helpful and responded to questions quickly. No problem too big or too small. Friendly teaching style meant I didn’t feel embarrassed to ask silly questions.
Louise
I like your jokes. It was always nice how you made us giggle sometimes. Your responsiveness was super appreciated and i loved the additional resources. I think it was nice that you spoke slowly and clearly
Elizaveta
First, Anuj makes efforts to simplify explanations of a concept and gives practical examples in a structured way. Second, he doesn’t just teach us things. He takes time to listen to our concerns and motivates us by sharing his personal experience and thoughts as an experienced software engineer.
Amanda Kartikasari
The presentations you prepare yourself are much more information dense, available and I found some topics are much easier to grasp using them, so grateful for the extra materials you always provide.
Asya Seagrave
Very thorough in terms of showing how to get the code to initially work and what the different errors could be, as well as how to fix them. Very efficient teaching style!
Cerys Cullen
Really liked your teaching style, always very clear and you explained things nice and simply which was great when learning a brand new topic! Loved your videos which were concise, easy to follow and super helpful for going back over to practice with. Loved all the extra resources you provided, your blog, and just in general how helpful and passionate you are about what you do! Thank you so much!
Megan Bradley
Anuj was a clear and concise instructor, who was always happy to help no matter the timing of it. I also liked that he made everyone feel welcome and fostered an inclusive culture, which made our team not afraid to present even if we were slightly unsure if our project work would live up the the standard of the rest of the group.
Deni Hancox
The style of the learning structure has been very constructive and the availabilityof the instructor helps to build confidence and security to try, fail and learn. 5 stars to the instructor style!
Maryl Duprat
Anuj was a fantastic instructor, super informative and knowledgeable. He was very approachable with questions, and clear and concise in his explanations. 🙂
Alice Pinch
Anuj is a great instructor who is very knowledgeable and passionate about what he teaches. He worked well with his co-instructor, which led to a positive learning experience. The course itself is an introductory course but still has a lot of material to cover, but Anuj was very meticulous in explaining concepts to ensure lessons were executed and understood. He is a great listener and always willing to answer questions no matter how obvious (and sometimes silly). I have already signed up for another course and am hoping he will be part of the team!
Pam
Well-structured lessons with the use of diagrams/images to help students understand concepts. Demonstrated examples step-by-step and answered any queries students had.
Maria
Anuj is a great instructor, who was incredibly dedicated to the course, both during teaching hours and after them. I really enjoyed the fun material he posted after the sessions, such as games and fun facts on our Slack channel. I highly appreciate that he was always responsive and patient with any queries or questions that I had, and so any confusions were very temporary.
Raimunda Bukartaite
Really engaging and helpful, always happy to answer any questions I had!
Muskaan
I really liked that the classes involved solving problems during the class and it wasn’t a person speaking alone all the time. I really appreciated the support and commitment of the instructors during the whole course.
Victoria Caballero
Instructions were really clear, and Anuj was very encouraging. He was always open to answering questions and very patient with the class.
Rashida Adekunle
Clear instructions and useful coding habits highlighted.
Asnath Lubanza
Always very helpful – going an extra mile to aid students. Always welcomes questions and will not make you feel bad or stupid for asking.
Maria
Patient when teaching, explains everything clearly and simply for non-techies(!), willing to go slower if us students find it fast or hard to keep up or understand, willing to go over topics and ensure everyone understands. Anuj goes above and beyond for the students despite understanding and learning differences. Outside of class and after class – a passionate and great teacher.
Ameera
Really enjoyed coding alongside you, clear and concise instructions and even some bits that were necessarily on the slides, but were SUPER helpful to us and our understanding of the language
Francessca
Very informative, supportive and friendly. The latter made you very approachable so I felt more at ease asking for help with things.
Amy Mulligan
I found the use cases made things very easy to follow and understand – when I was learning by myself, I was confused at some of the concepts because I couldn’t see how they would be applied, whereas you gave real-life examples that really helped it slot together!
Anastasija Medvedeva
Very supportive, very clear and gives great demonstrations and examples. The extra slides and YouTube videos were also a huge help for me.
Daisy Dobson
I liked that you walked through what you were writing for the code and showed different expamples for the same concept
Fabiha Chowdhury
Used relevant examples of code in the real world, and took the time to explain difficult ideas.
Chloe
I like you are very helpful and teaching the things again and again and make people comfortable to ask you a questions.
Pinar Seker
I think you taught everything really well, thank you! All questions you answered concisely and shared lots of resources.
Ria Kakkad
Your words were organize, not chaotic. You know how to pass the knowledge to students. Thank you
JL
Very clear speaker. Passionate about what you are speaking about. Add your own knowledge into the classes and don’t just read from the script/slides. Explain things in an easy to understand manner. Always available to help.
Caroline Bell
Great teacher, extremely helpful and taught the course in a way which was understandable
Emma McKinney
I liked it, it was very helpful with the tips and notes, also the knowledge was definitely evident so was trustworthy and made me feel comfortable when I thought I asked a stupid question and always had help available.
Sermin Efendi
Really clear, concise and supportive. Broke down complicated problems easily with other examples. The extra content you created with your slides were incredibly helpful.
Nalani St Louis
lots of personality and availability to answer questions/doubts
Liyaan Khoso
Everything Anuj! As a teacher myself, I loved learning from you. You were so patient with us and answered every question we asked so well. You also had a way of making difficult things seem easy. Your passion and dedication showed.
Onyeoma Adigwe
very structured and easy to follow. Very supportive with all the students and always giving insightful advices.
Miriem Shaimi
I really like how you try to simplify and give real-life analogies to coding concepts. Really grateful for all the support you give to us students, especially by addressing our questions and for being available when we are lost. Really grateful for it as it shows how much you care for your students.
Kathleen
Clear lessons, easy to follow even for a beginner. Very knowledgeable and gives great encouragement to beginner coders. Really helpful and responded to questions quickly. No problem too big or too small. Friendly teaching style meant I didn’t feel embarrassed to ask silly questions.
Louise
I liked Anuj’s style of teaching, really clear and easy to understand!
Carolina Opazo
The style of the learning structure has been very constructive and the availabilityof the instructor helps to build confidence and security to try, fail and learn. 5 stars to the instructor style!
Maryl Duprat
Anuj was a fantastic instructor, super informative and knowledgeable. He was very approachable with questions, and clear and concise in his explanations. 🙂
Alice Pinch
Anuj is a great instructor who is very knowledgeable and passionate about what he teaches. He worked well with his co-instructor, which led to a positive learning experience. The course itself is an introductory course but still has a lot of material to cover, but Anuj was very meticulous in explaining concepts to ensure lessons were executed and understood. He is a great listener and always willing to answer questions no matter how obvious (and sometimes silly). I have already signed up for another course and am hoping he will be part of the team!
Pam
Well-structured lessons with the use of diagrams/images to help students understand concepts. Demonstrated examples step-by-step and answered any queries students had.
Maria
Anuj is a great instructor, who was incredibly dedicated to the course, both during teaching hours and after them. I really enjoyed the fun material he posted after the sessions, such as games and fun facts on our Slack channel. If I were to give a piece of constructive advice, I would recommend slowing down a little bit with the course material. For us newbies it can sometimes be difficult to keep up. That being said, I highly appreciate that he was always responsive and patient with any queries or questions that I had, and so any confusions were very temporary. Slowing the pace down a bit would be the cherry on top!
Raimunda Bukartaite
Really engaging and helpful, always happy to answer any questions I had!
Muskaan
Overall very good and in depth teaching. Sometimes more explanation would be better, maybe slow down when showing examples.
Dominika
I really liked that the classes involved solving problems during the class and it wasn’t a person speaking alone all the time. I really appreciated the support and commitment of the instructors during the whole course.
Victoria Caballero
I thought the instructions were really clear and the instructor was very encouraging. They were always open to answering questions and very patient with the class. I think the lesson would have benefitted from more “teacher persona” tactics to get the class more involved with their cameras on and answering questions so that the lesson would have a more interactive feel.
Rashida Adekunle
Clear instructions and useful coding habits highlighted.
Asnath Lubanza
Always very helpful – going an extra mile to aid students. Always welcomes questions and will not make you feel bad or stupid for asking.
Maria
First, Anuj makes efforts to simplify explanations of a concept and gives practical examples in a structured way. Second, he doesn’t just teach us things. He takes time to listen to our concerns and motivates us by sharing his personal experience and thoughts as an experienced software engineer.
Amanda Kartikasari
Patient when teaching, explains everything clearly and simply for non-techies(!), willing to go slower if us students find it fast or hard to keep up or understand, willing to go over topics and ensure everyone understands. Anuj goes above and beyond for the students despite understanding and learning differences. Outside of class and after class – a passionate and great teacher.
Ameera
Really enjoyed coding alongside you, clear and concise instructions and even some bits that were necessarily on the slides, but were SUPER helpful to us and our understanding of the language
Francessca
Very informative, supportive and friendly. The latter made you very approachable so I felt more at ease asking for help with things.
Amy Mulligan
I found the usecases made things very easy to follow and understand – when I was learning by myself, I was confused at some of the concepts because I couldn’t see how they would be applied, whereas you gave real-life examples that really helped it slot together!
Anastasija Medvedeva
confident and helpful
louise
Anuj was a clear and concise instructor, who was always happy to help no matter the timing of it. I also liked that he made everyone feel welcome and fostered an inclusive culture, which made our team not afraid to present even if we were slightly unsure if our project work would live up the the standard of the rest of the group.
Deni Hancox
Very supportive, very clear and gives great demonstrations and examples. The extra slides and YouTube videos were also a huge help for me.
Daisy Dobson
Very thorough in terms of showing how to get the code to initially work and what the different errors could be, as well as how to fix them. Very efficient teaching style!
Cerys Cullen
I liked that you walked through what you were writing for the code and showed different expamples for the same concept
Fabiha Chowdhury
Used relevant examples of code in the real world, and took the time to explain difficult ideas.
Chloe
I like you are very helpful and teaching the things again and again and make people comfortable to ask you a questions.
Pinar Seker
I think you taught everything really well, thank you! All questions you answered concisely and shared lots of resources.
Ria Kakkad
Your words were organize, not chaotic. You know how to pass the knowledge to students. Thank you
JL
Very clear speaker. Passionate about what you are speaking about. Add your own knowledge into the classes and don’t just read from the script/slides. Explain things in an easy to understand manner. Always available to help.
Caroline Bell
Great teacher, extremely helpful and taught the course in a way which was understandable
Emma McKinney
I liked it, it was very helpful with the tips and notes, also the knowledge was definitely evident so was trustworthy and made me feel comfortable when I thought I asked a stupid question and always had help available.
Sermin Efendi
Well researched resources. Easy explanation. Great examples.
Sefat
Really clear, concise and supportive. Broke down complicated problems easily with other examples. The extra content you created with your slides were incredibly helpful.
Nalani St Louis
lots of personality and availability to answer questions/doubts
Liyaan Khoso
Everything Anuj! As a teacher myself, I loved learning from you. You were so patient with us and answered every question we asked so well. You also had away of making difficult things seem easy. Your passion and dedication showed.
Onyeoma Adigwe
The presentations you prepare yourself are much more information dense, available and I found some topics are much easier to grasp using them, so grateful for the extra materials you always provide.
Asya Seagrave
very structured and easy to follow. Very supportive with all the students and always giving insightful advices.
Miriem Shaimi
I really like how you try to simplify and give real-life analogies to coding concepts. Really grateful for all the support you give to us students, especially by addressing our questions and for being available when we are lost. Really grateful for it as it shows how much you care for your students.